The differences between HTML and CSS.
When talking about building a website the core languages used are HTML and CSS. They are written out as code which our web browsers translate and then displays. A website can be created with just HTML but with the use of CSS or Cascading Style Sheets we can improve the overall look and feel of our website.
If we think of HTML as the sketch or markup of a website and the CSS as the styling, we can take the process of a painting a picture into account and how it is formed as an analogy. We first use HTML to dictate the content and structure of our webpage just as an artist would sketch what they are about to paint. They outline the painting in pencil giving them the structure and content. Once we are happy with our
HTML we then need to add styling to introduce the display and design of the site. We utilize CSS to apply this styling to our exsisting HTML elements/content. Our artist at this stage would then apply paint to their sketch bringing life and colour
to the image, using different brush strokes and textures and brush width to complete and make their product more appealing.
Control Flow and loops
As in all languages there is a dictated flow on how we read and interpert the content, the same applies to our code and how it is read and executed. Just like a can of pringles (original flavour naturally) we start at the top and work our way down. We can think of each pringle as a line of code thats gets read and executed in succssesion.
Uh oh..
What happens when we reach the end of our can of pringles?!? This is where loops can come in to play
Javascript is another language we use and it is used for the interactivity and functionality of a website. Through this language we can implement what is called loops to execute a block of code repeatedly until we met certain conditions based on if the condition is true or false. In certain cases we can use loops to manipulate our control flow thus changing the flow of execution.
For instance using our pringles analogy lets take a look at how we can dictate how we eat our chips using our loops in Javascript.
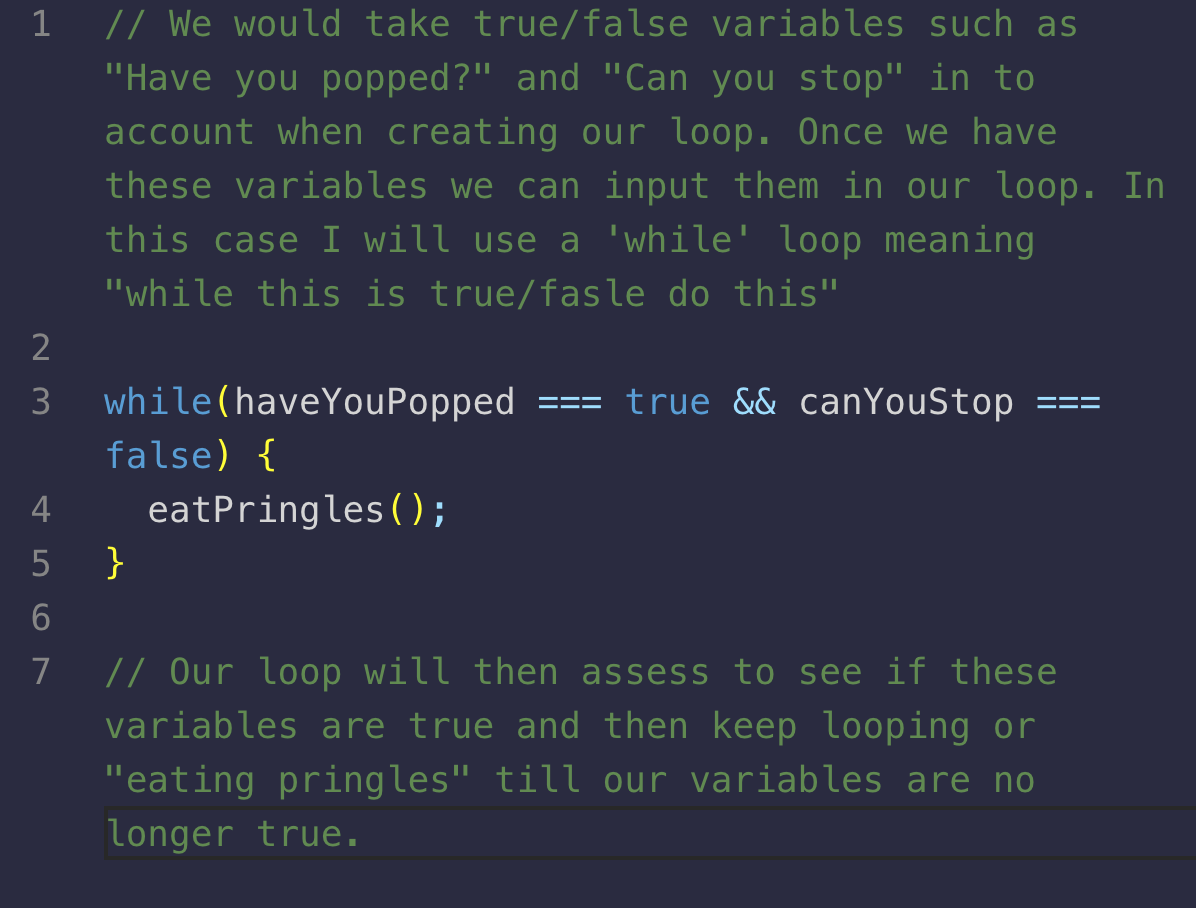
So now we know HTML defines the structure, CSS provides the appearance/styling and JavaScript deals with interactivity. The glue that holds it all together is the DOM Document Object Module
The DOM is an entire representation of the HTML and CSS content. It is an object-oriented representation of the web page, which can be modified with a scripting language such as JavaScript.
The formal name for everything we see in the DOM is called nodes. Elements, attributes, text content, comments, document related stuff etc are all referred to as nodes. By giving everything a object representation we can access it using Javascript. All of the properties, methods, and events available for manipulating and creating web pages are organized into objects. Bear in mind the DOM is not a programming language, but without it, the JavaScript language wouldn't have any model or notion of web pages. So by understanding how the DOM is represented allows us to have a better grasp of where our elements lie within a webpage and how we can access them.
Arrays and Objects
When storing data into groups we can use two different collections types that serve two different purposes. Arrays, and objects. We can use arrays when we have a series of variables that we want to store and retrieve under a single variable where the order matters, usually its the same type of thing because that selection of data is intended to be held together for whatever reason, and we can access the data by using whats called its index. But if we wanted to store many things under a single indentifier this is where we would use an object. An object would represent a thing that has multiple properties for example a drivers licence which holds multiple characterstics. Name, date of birth, drivers licence version, expiry date, donor etc, in objects we call these properties and each property consists of a key:value pair. In our example the "Name" being the key and my name "David" being the value. So objects are useful when we want to store and retrieve multiple types of properties where the order doesnt matter.
Functions
In JavaScript we can build a block of code that makes something do a task. Sort of like a procedure or routine that will be carried out when we "invoke" it, this can be done automatically, or from an event occuring like the click of a mouse button, or it can be called within the code itself.
A function is composed of a function name which is used to invoke the function, parameters which become values when we invoke the function, we call the values arguments which can be passed into our function and used within the function, the statement or "body" of the function which declares its designated task and usually a return value, which will return whatever it is that you passed in after its executed the task.